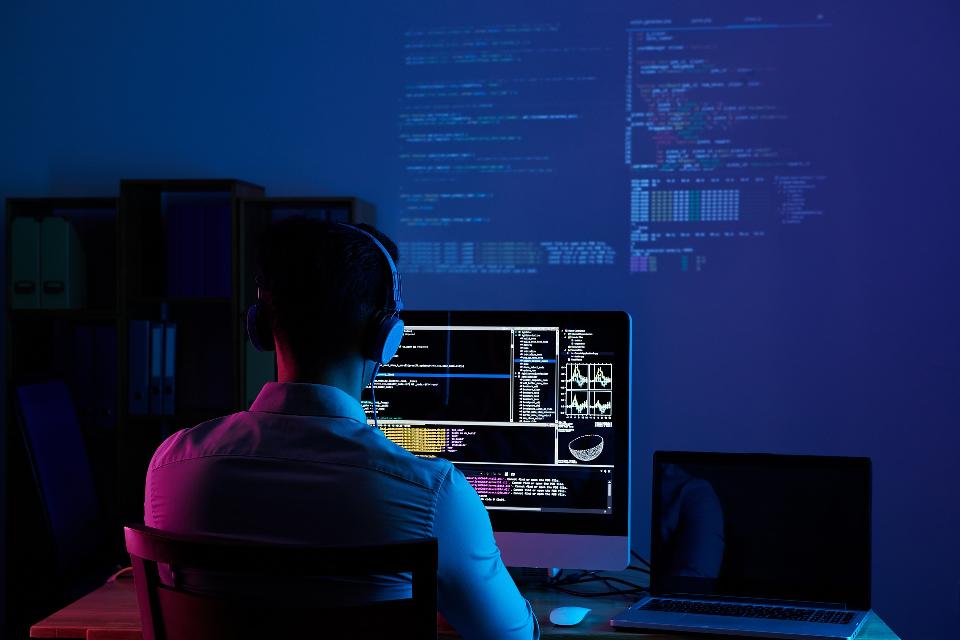
#bootcamp
#python
In this chapter, we dive into the code and explore the implementation of the game step by step.
First, we will define a list of questions as dictionaries. Each sub-dictionary in the dictionary contains the question, the available options as a single string with a letter for answers, and the correct letter answer field we will use to check the input of the user.
Then we create Game class, which takes the player's name and the list of questions as parameters. Inside the class, we have created methods like menu, start, and query_questions to handle the game's logic.
The menu method allows the player to start a new game or exit the program after the initial game has ended. It prompts the user for input and checks that only valid choices are accepted.
The start method initializes the player's score to zero value and calls the query_question method to begin the quiz. Acting as a restart method as well.
The query_questions method iterates through each question. After the user has answered it checks if the answer is correct and updates the player's score.
In the end, the game displays the score. Then the user is prompted with a menu method to offer the option of playing another round or exiting the game.
By creating this game, we reinforce our understanding of previous chapters and introduced how Python deals with inputs. I/O you can understand input() as the input method, and print() as the output method of taking in and representing data in a console or other medium.
You can try to create a more complex menu, maybe even a scoreboard. Think about what type of data you would use for the scoreboard. A list, dictionary, concatenate string? Maybe a text file to create persistent storage for scores? We still did not use files in Python, we will go over them in the next chapter. But it is not too complicated and you can try to find out how to do it on your own.
You could even create more functions and divide query_questions into more functions and have a divided method for reading the dictionary and a separate method to check the outcome of user choices.
questions = [
{
"question": "Question 1: In the movie 'The Matrix,' what is the name of the hacker who later becomes 'Neo'?",
"options": "a) Trinity, b) Morpheus, c) Neo, d) Cipher",
"answer": "c"
},
{
"question": "Question 2: Which movie features a group of hackers who plan a bank heist using a worm called 'Hydra'?",
"options": "a) Swordfish, b) Hackers, c) Live Free or Die Hard, d) The Italian Job",
"answer": "a"
},
{
"question": "Question 3: In the movie 'Ocean's Eleven,' what is the name of the character played by George Clooney?",
"options": "a) Danny Ocean, b) Rusty Ryan, c) Linus Caldwell, d) Terry Benedict",
"answer": "a"
},
{
"question": "Question 4: Which film portrays the story of a high school student who hacks into a supercomputer and nearly triggers a nuclear war?",
"options": "a) WarGames, b) Sneakers, c) The Social Network, d) Blackhat",
"answer": "a"
},
{
"question": "Question 5: What is the name of the computer-generated character in the movie 'Tron'?",
"options": "a) Flynn, b) Sark, c) Tron, d) Clu",
"answer": "c"
},
{
"question": "Question 6: Which movie involves a group of thieves who plan to steal a mysterious black box from a heavily guarded corporation?",
"options": "a) Inception, b) The Net, c) Antitrust, d) Sneakers",
"answer": "d"
},
{
"question": "Question 7: In the film 'The Social Network,' what is the name of the social networking website created by Mark Zuckerberg?",
"options": "a) Facebook, b) MySpace, c) Twitter, d) Instagram",
"answer": "a"
},
{
"question": "Question 8: Which movie features a team of illusionists who use their skills to pull off a series of heists?",
"options": "a) Now You See Me, b) The Prestige, c) Ocean's Eleven, d) The Town",
"answer": "a"
},
{
"question": "Question 9: In the movie 'The Imitation Game,' which mathematician and computer scientist is portrayed by Benedict Cumberbatch?",
"options": "a) Alan Turing, b) Steve Jobs, c) Bill Gates, d) Nikola Tesla",
"answer": "a"
},
{
"question": "Question 10: Which film revolves around a software engineer who discovers a hidden function in a popular video game?",
"options": "a) Ready Player One, b) Ex Machina, c) The Matrix, d) Tron: Legacy",
"answer": "b"
},
]
class Game:
def __init__(self, player, questions):
self.player = player
self.questions = questions
self.start()
def menu(self, attemtp=False):
if attemtp:
print("Must be 'y' or 'n'")
user = input("New Game y/n:").lower()
print(user)
if user == "y":
self.start()
elif user == "n":
exit()
else:
self.menu(True)
def start(self):
self.score = 0
self.query_questions()
def query_questions(self):
for i in self.questions:
print("Question {}: \n{}\n".format(i['question'], i['options']), end="")
user = input("Enter answer: ")
if user.lower() == i["answer"]:
print("Correct Answer")
self.score += 1
else:
print("Wrong Answer")
print("{}\nYour score is {}/{}".format(self.player, self.score, len(self.questions)))
self.menu()
obj = Game(input("Enter your name and press ENTER:"), questions )
[root@techtoapes]$ Author Luka
Login to comment.